티스토리 뷰
반응형
문제 링크
Permutations - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
문제
Given an array nums of distinct integers, return all the possible permutations. You can return the answer in any order.
Example 1:
Input: nums = [1,2,3] Output: [[1,2,3],[1,3,2],[2,1,3],[2,3,1],[3,1,2],[3,2,1]]
Example 2:
Input: nums = [0,1] Output: [[0,1],[1,0]]
Example 3:
Input: nums = [1] Output: [[1]]
Constraints:
- 1 <= nums.length <= 6
- -10 <= nums[i] <= 10
- All the integers of nums are unique.
문제 풀이
이 문제는 주어진 정수들로 만들 수 있는 모든 순열을 구하는 문제입니다.
똑같은 값이 여러번 들어가면 안되기 때문에 중복체크를 해줘야하는게 가장 중요한 아이디어 입니다.
일반적인 백트래킹을 수행하는데, 이미 사용한 숫자인지에 대한 체크만 처리해주시면 됩니다.
그리고 return 한 뒤에는 방문여부를 초기화 해줘야 모든 순열을 구할 수 있습니다!
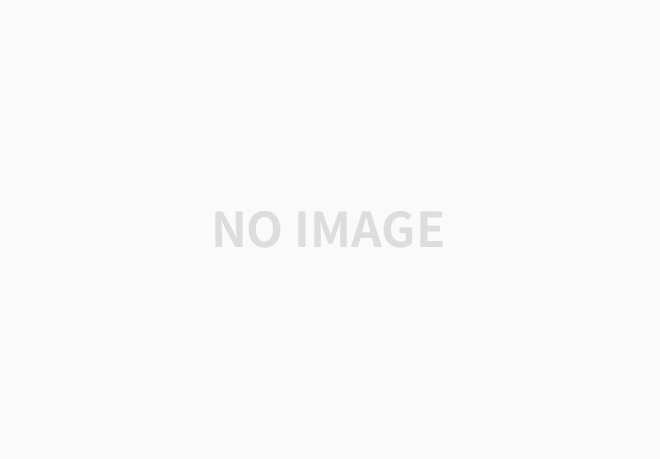
소스 코드
class Solution {
func permute(_ nums: [Int]) -> [[Int]] {
var result: [[Int]] = []
// 중복을 허용하지 않게 만들어야 하므로 아래와 같이 사용여부를 체크해줍니다.
var visited: [Bool] = [Bool](repeating: false, count: nums.count)
func permutation(_ nowPermute: [Int]) {
// 만약 현재 순열의 원소 수가 주어진 원소수와 같다면 결과에 추가!
if nowPermute.count == nums.count {
result.append(nowPermute)
return
}
for i in 0..<nums.count {
// 이미 사용한 값이라면 넘어갑니다.
if visited[i] {
continue
} else {
visited[i] = true
permutation(nowPermute + [nums[i]])
visited[i] = false
}
}
}
permutation([])
return result
}
}
반응형
'Algorithm > LeetCode' 카테고리의 다른 글
[LeetCode] 62번 - Unique Paths [Swift] (0) | 2021.02.01 |
---|---|
[LeetCode] 56번 - Merge Intervals [Swift] (0) | 2021.02.01 |
[LeetCode] 39번 - Combination Sum [Swift] (0) | 2021.01.27 |
[LeetCode] 53번 - Maximum Subarray [Swift] (0) | 2021.01.22 |
[LeetCode] 34번 - Find First and Last Position of Element in Sorted Array [Swift] (0) | 2021.01.22 |
공지사항
최근에 올라온 글
최근에 달린 댓글
- Total
- Today
- Yesterday
링크
TAG
- design
- operating
- Combine
- operator
- 코딩테스트
- 테이블뷰
- 프로그래밍
- BFS
- System
- dfs
- pattern
- Publisher
- 아이폰
- 앱개발
- Xcode
- document
- Swift
- 문법
- 스위프트
- 코테
- 알고리즘
- DP
- mac
- OS
- Apple
- OSTEP
- 동시성
- 백준
- 자료구조
- IOS
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
글 보관함